- 81浏览
- 2022-10-09
注意:你需要在OpenAI网站上注册并获取API密钥。
<!DOCTYPE html>
<html>
<head>
<title>Chat GPT</title>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<script src="https://cdn.jsdelivr.net/npm/axios/dist/axios.min.js"></script>
<style type="text/css">
body {
margin: 0;
padding: 0;
font-family: Arial, sans-serif;
background-color: #f7f7f7;
}
.container {
width: 100%;
max-width: 600px;
margin: 0 auto;
padding: 20px;
box-sizing: border-box;
background-color: #fff;
border-radius: 5px;
box-shadow: 0px 0px 10px #ddd;
}
h1 {
margin: 0;
font-size: 24px;
font-weight: bold;
text-align: center;
}
.chatbox {
height: 400px;
overflow-y: scroll;
margin-bottom: 10px;
padding: 10px;
box-sizing: border-box;
background-color: #f7f7f7;
border-radius: 5px;
box-shadow: inset 0px 0px 10px #ddd;
}
.inputbox {
display: flex;
flex-wrap: wrap;
align-items: center;
margin-bottom: 10px;
}
.inputbox input[type="text"] {
flex-grow: 1;
padding: 10px;
box-sizing: border-box;
background-color: #fff;
border: none;
border-radius: 5px;
box-shadow: 0px 0px 10px #ddd;
font-size: 16px;
margin-right: 10px;
}
.inputbox button[type="submit"] {
padding: 10px;
box-sizing: border-box;
background-color: #007bff;
border: none;
border-radius: 5px;
color: #fff;
text-transform: uppercase;
font-size: 16px;
font-weight: bold;
cursor: pointer;
transition: background-color 0.3s ease;
-webkit-transition: background-color 0.3s ease;
-moz-transition: background-color 0.3s ease;
-o-transition: background-color 0.3s ease;
}
.inputbox button[type="submit"]:hover {
background-color: #0062cc;
}
</style>
</head>
<body>
<div class="container">
<h1>Chat GPT</h1>
<div class="chatbox" id="chatbox"></div>
<form id="chatForm" onsubmit="return sendMessage()">
<div class="inputbox">
<input type="text" name="message" id="message" placeholder="Type your message here...">
<button type="submit">Send</button>
</div>
</form>
</div>
<script type="text/javascript">
const api_key = 'YOUR_API_KEY';
const api_url = 'https://api.openai.com/v1/';
function sendMessage() {
event.preventDefault();
const messageInput = document.getElementById('message');
const message = messageInput.value;
if (message != '') {
displayMessage(message, 'user');
messageInput.value = '';
axios.post(api_url + 'engines/davinci-codex/completions', {
prompt: message,
max_tokens: 150,
n: 1,
stop: ['\n']
}, {
headers: {
'Content-Type': 'application/json',
'Authorization': 'Bearer ' + api_key
}
})
.then(response => {
const botMessage = response.data.choices[0].text.trim();
displayMessage(botMessage, 'bot');
})
.catch(error => console.log(error));
}
return false;
}
function displayMessage(message, userType) {
const chatbox = document.getElementById('chatbox');
const messageWrapper = document.createElement('div');
const messageBubble = document.createElement('div');
messageBubble.textContent = message;
messageBubble.classList.add('message-bubble');
messageWrapper.classList.add('message-wrapper');
messageWrapper.classList.add(userType);
messageWrapper.appendChild(messageBubble);
chatbox.appendChild(messageWrapper);
chatbox.scrollTop = chatbox.scrollHeight;
}
</script>
</body>
</html>
解释:首先,我们引入了Axios库来进行API调用。
<script src="https://cdn.jsdelivr.net/npm/axios/dist/axios.min.js"></script>
在script标签中,我们定义了API密钥和API URL。
const api_key = 'YOUR_API_KEY';
const api_url = 'https://api.openai.com/v1/';
请将YOUR_API_KEY替换为你在OpenAI网站上获取的API密钥。
然后,我们定义了发送消息的函数sendMessage()。
function sendMessage() {
event.preventDefault();
const messageInput = document.getElementById('message');
const message = messageInput.value;
if (message != '') {
displayMessage(message, 'user');
messageInput.value = '';
axios.post(api_url + 'engines/davinci-codex/completions', {
prompt: message,
max_tokens: 150,
n: 1,
stop: ['\n']
}, {
headers: {
'Content-Type': 'application/json',
'Authorization': 'Bearer ' + api_key
}
})
.then(response => {
const botMessage = response.data.choices[0].text.trim();
displayMessage(botMessage, 'bot');
})
.catch(error => console.log(error));
}
return false;
}
这个函数会首先阻止表单的默认行为,然后获取用户输入的消息,调用displayMessage()函数在聊天界面上显示用户发送的消息,并将这个消息作为prompt参数发送给OpenAI的API。我们使用Axios库来发送POST请求,并将API密钥和Content-Type设置为请求header的一部分。API返回的是一个包含一条消息的数组对象,我们取出这条消息并调用displayMessage()函数在聊天界面上显示。
我们还定义了一个displayMessage()函数,用于在聊天框中显示发送和接收的消息。
function displayMessage(message, userType) {
const chatbox = document.getElementById('chatbox');
const messageWrapper = document.createElement('div');
const messageBubble = document.createElement('div');
messageBubble.textContent = message;
messageBubble.classList.add('message-bubble');
messageWrapper.classList.add('message-wrapper');
messageWrapper.classList.add(userType);
messageWrapper.appendChild(messageBubble);
chatbox.appendChild(messageWrapper);
chatbox.scrollTop = chatbox.scrollHeight;
}
这个函数创建了一个包含消息的div元素,并将其添加到聊天框中。我们还通过给这个div元素添加不同的类名来区分是用户还是机器人发送的消息。最后,我们将聊天框的滚动条移动到底部,以便用户始终能够看到最新的消息。 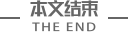
版权声明:
1、本文系转载,版权归原作者所有,旨在传递信息,不代表看本站的观点和立场。
2、本站仅提供信息发布平台,不承担相关法律责任。
3、若侵犯您的版权或隐私,请联系本站管理员删除。
4、本文由会员转载自互联网,如果您是文章原创作者,请联系本站注明您的版权信息。