- 58浏览
- 2022-10-25
首先,我们需要在数据库中创建一个用于存储评论信息的数据表。可以使用如下的 SQL 语句创建一个名为 comments 的表:
CREATE TABLE `comments` (
`id` int(11) NOT NULL AUTO_INCREMENT,
`content` varchar(255) NOT NULL,
`created_at` timestamp NOT NULL DEFAULT CURRENT_TIMESTAMP,
PRIMARY KEY (`id`)
) ENGINE=InnoDB DEFAULT CHARSET=utf8;
创建控制器
创建一个名为 Comment 的控制器,代码如下:
<?php
namespace app\index\controller;
use think\Controller;
use think\Request;
use app\index\model\Comment as CommentModel;
class Comment extends Controller
{
public function index()
{
$list = CommentModel::all();
$this->assign('list', $list);
return $this->fetch();
}
public function add(Request $request)
{
if ($request->isPost()) {
$data = $request->post();
$result = $this->validate($data, 'Comment');
if (true !== $result) {
return ['error' => $result];
}
$comment = new CommentModel();
$comment->content = $data['content'];
$comment->save();
return ['msg' => '评论成功'];
}
}
}
创建模型
创建一个名为 Comment 的模型,代码如下:
<?php
namespace app\index\model;
use think\Model;
class Comment extends Model
{
protected $autoWriteTimestamp = true;
protected $insert = ['created_at'];
}
创建验证器
创建一个名为 Comment 的验证器,用于验证提交的评论内容是否合法。代码如下:
<?php
namespace app\index\validate;
use think\Validate;
class Comment extends Validate
{
protected $rule = [
'content' => 'require|max:255',
];
protected $message = [
'content.require' => '评论内容不能为空',
'content.max' => '评论内容不能超过255个字符',
];
}
创建路由
在 application/route.php 文件中添加以下路由配置:
Route::get('comment', 'index/comment/index');
Route::post('comment/add', 'index/comment/add');
完整代码
控制器:application/index/controller/Comment.php
<?php
namespace app\index\controller;
use think\Controller;
use think\Request;
use app\index\model\Comment as CommentModel;
class Comment extends Controller
{
public function index()
{
$list = CommentModel::all();
$this->assign('list', $list);
return $this->fetch();
}
public function add(Request $request)
{
if ($request->isPost()) {
$data = $request->post();
$result = $this->validate($data, 'Comment');
if (true !== $result) {
return ['error' => $result];
}
$comment = new CommentModel();
$comment->content = $data['content'];
$comment->save();
return ['msg' => '评论成功'];
}
}
}
模型:application/index/model/Comment.php
<?php
namespace app\index\model;
use think\Model;
class Comment extends Model
{
protected $autoWriteTimestamp = true;
protected $insert = ['created_at'];
}
验证器:application/index/validate/Comment.php
<?php
namespace app\index\validate;
use think\Validate;
class Comment extends Validate
{
protected $rule = [
'content' => 'require|max:255',
];
protected $message = [
'content.require' => '评论内容不能为空',
'content.max' => '评论内容不能超过255个字符',
];
}
路由:application/route.php
<?php
use think\Route;
Route::get('comment', 'index/comment/index');
Route::post('comment/add', 'index/comment/add');
注意事项:
代码中的路由、控制器、模型和验证器命名空间均为 app\index,请根据自己的实际情况进行修改。
add() 方法接收 POST 请求,并使用验证器对请求参数进行验证。
验证器的规则和消息可以根据实际情况进行修改。
Comment 模型设置了自动写入时间戳和插入时自动设置 created_at 字段。
add() 方法返回 JSON 格式数据,可根据需要进行修改。
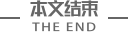
版权声明:
1、本文系转载,版权归原作者所有,旨在传递信息,不代表看本站的观点和立场。
2、本站仅提供信息发布平台,不承担相关法律责任。
3、若侵犯您的版权或隐私,请联系本站管理员删除。
4、本文由会员转载自互联网,如果您是文章原创作者,请联系本站注明您的版权信息。